Learn how to transform document creation, editing, and organization with the Google Docs API. Gain the skills to automate generating documents, extracting content, working together effortlessly, and connecting with other Google Workspace apps. This exhaustive manual offers developers and businesses practical examples, best practices, and troubleshooting tips.
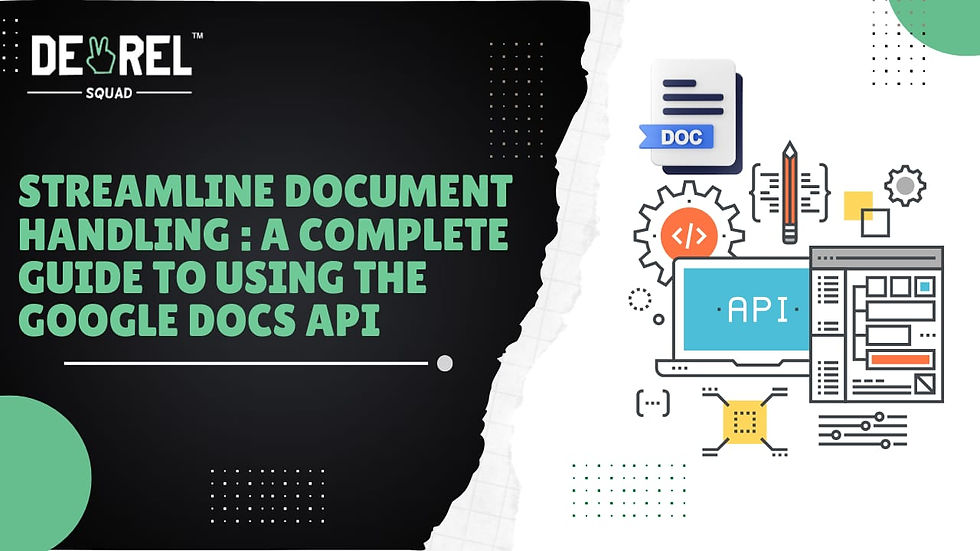
Overview
Efficient document management is essential for businesses, educators, and developers in today's fast-moving digital era. The Google Docs API, a necessary part of the Google Cloud Platform, allows users to automate and simplify document-related tasks. This guide will act as a comprehensive manual for utilizing the Google Docs API, highlighting its features and showing how to incorporate it into your work process for optimal efficiency.
What is the Google Docs API?
The Google Docs API enables developers to generate, access, and edit Google Docs files automatically. It provides a method to automate tasks, connect with other apps, and create personalized workflows. The API allows for document management, inserting and formatting text, and manipulating embedded objects such as images and tables.
Getting Started with the Google Docs API
Set Up Your Google Cloud Project
The initial step in utilizing the API is setting up a Google Cloud project and activating the Google Docs API.
Create a New Project: Head to the Google Cloud Console, initiate a new project and assign it a name.
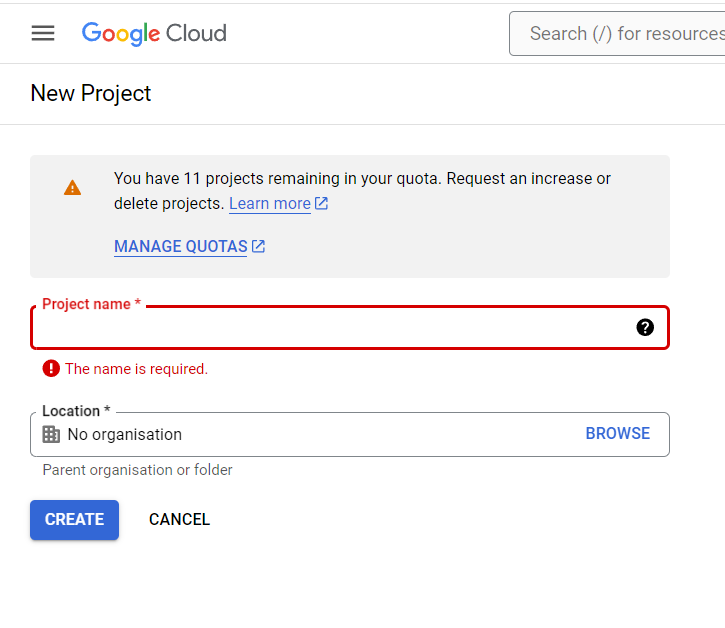
Enable the Google Docs API: Activate the Google Docs API by accessing the Cloud Console, going to "APIs & Services" > "Library", and looking up the "Google Docs API". Please select it and activate the API for your project.

Create Credentials: To verify your requests, you must create credentials. Navigate to "APIs & Services" > "Credentials", press "Create Credentials", and choose "Service Account".
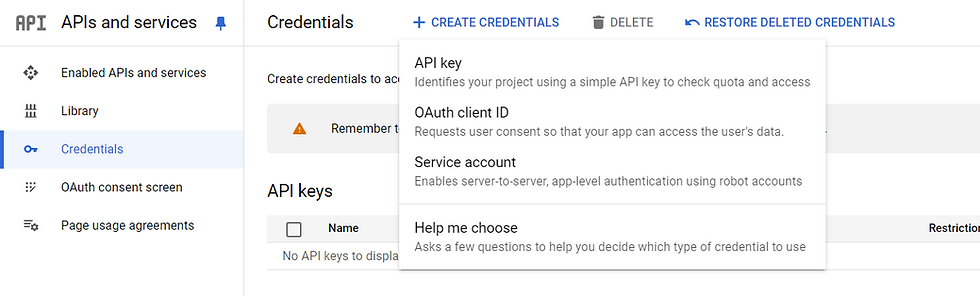
Setting Up Your Environment
A programming environment is required to utilize the Google Docs API. This tutorial uses Python, but the API is compatible with various languages, such as JavaScript, Java, and Go.
1. Install the Google Client Library:
pip install google-auth google-auth-oauthlib google-auth-httplib2 google-api-python-client
2. Authenticate and Initialize the Service:
from google.oauth2 import service_account
from googleapiclient.discovery import build
SCOPES = ['https://www.googleapis.com/auth/documents']
SERVICE_ACCOUNT_FILE = 'path/to/your/service-account-file.json'
credentials = service_account.Credentials.from_service_account_file(
SERVICE_ACCOUNT_FILE, scopes=SCOPES)
service = build('docs', 'v1', credentials=credentials)
Creating a New Document
To create a new document using the Google Docs API:
document = service.documents().create(body={'title': 'New Document'}).execute()
document_id = document.get('documentId')
print(f'Document ID: {document_id}')
Reading a Document
To read a document with the Google Docs API:
document = service.documents().get(documentId=document_id).execute()
print(f'Title: {document.get("title")}')
print('Content:')
for element in document.get('body').get('content'):
print(element.get('paragraph').get('elements')[0].get('textRun').get('content'))
Modifying a Document
To insert text into a document using the Docs API Guide:
requests = [
{
'insertText': {
'location': {
'index': 1,
},
'text': 'Hello, world!'
}
}
]
result = service.documents().batchUpdate(documentId=document_id, body={'requests': requests}).execute()
Formatting Text
You can apply various text styles using the Google Docs API:
requests = [
{
'updateTextStyle': {
'range': {
'startIndex': 1,
'endIndex': 13,
},
'textStyle': {
'bold': True,
'italic': True,
},
'fields': 'bold,italic'
}
}
]
result = service.documents().batchUpdate(documentId=document_id, body={'requests': requests}).execute()
Why Use Google Docs API?
Collaborating in Real-Time
The Google Docs API enables live collaboration. It allows you to distribute documents to numerous users and programmatically control access permissions. This is especially beneficial for teams collaborating on documents together.
Integrating with Other APIs
Combine the Google Docs API with other Google Workspace tools like Google Drive for complete document management solutions. For instance, streamline uploading documents to Google Drive once created.
Error Handling
Implementing error handling is crucial when utilizing the Google Docs API to address potential problems like rate limits and API quota restrictions.
try:
result = service.documents().batchUpdate(documentId=document_id, body={'requests': requests}).execute()
except HttpError as err:
print(f'An error occurred: {err}')
Conclusion
The Google Docs API is a robust tool for automating and improving document management. Whether making new documents, editing and viewing existing ones or connecting with other services, the Google Docs API provides various features to make your work more efficient. Using the Google Docs API, you can streamline processes, decrease manual labour, and develop document management solutions that are more effective and can grow with your needs.
FAQs
1. What is the Google Docs API?
Developers can use the Google Docs API to automatically perform tasks and connect with other applications by programmatically generating, accessing, and editing Google Docs documents.
2. How do I get started with the Google Docs API?
Create a Google Cloud Platform account, activate the Google Docs API, and generate OAuth 2.0 credentials. Next, set up the required Google client libraries and authorize your application.
3. Can I use the Google Docs API to collaborate in real time?
Yes, the Google Docs API enables simultaneous collaboration, permitting the sharing of documents with numerous users and the automated management of access permissions.
4. What programming languages are supported by the Google Docs API?
The Google Docs API supports multiple programming languages, such as Python, JavaScript, Java, and Go.
5. Can I integrate the Google Docs API with other Google services?
Combining the Google Docs API with other Google Workspace tools like Google Drive allows you to develop complete document management solutions that automate tasks such as uploading generated documents.
References:
Feel free to use the reference links for more detailed information and guides: Google Docs API
Check out the GitHub repository for detailed source code and functional examples. Explore practical implementations to enhance your projects.
Comments