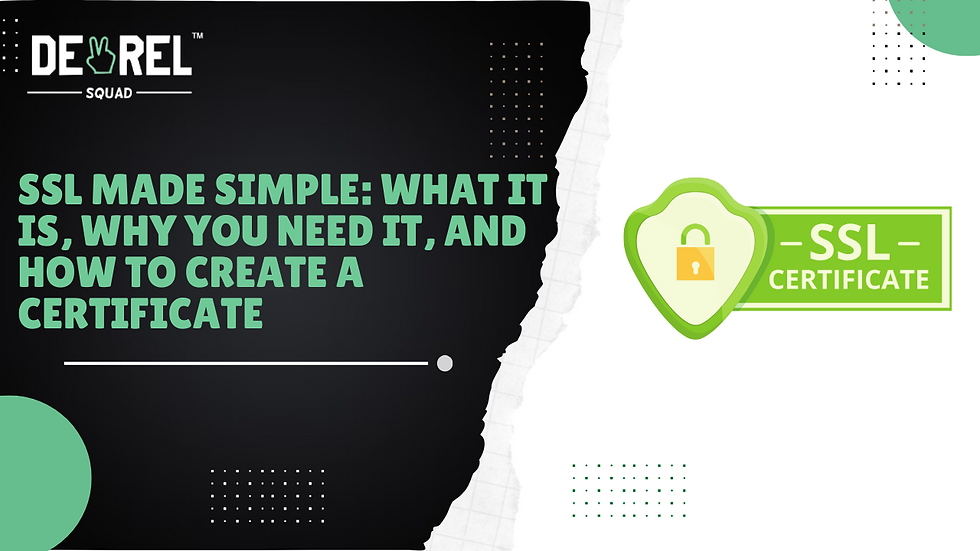
We are using SSL(Secure Socket Layer) to secure communication on the Internet. It encrypts communication between two nodes: a web browser and a web server. No one can decrypt it later; if they try, it will take thousands of years.
So, how do we encrypt data, and what do we use to encrypt it? Later, we will discuss how this is being implemented in SSL Certificates.
You can get the whole source code for this article here.
Firstly, why is encryption needed?
Let's suppose a browser sends critical data in plain text to a web server like passwords, net banking pin, credit card details or something else it is in plain text Anyone on the network can see that message content; instead, they can change it too, and this is called a man in the middle attack. Using different kinds of encryption techniques, we can prevent this:
How does encryption work?
Before diving into SSL, we must understand its two fundamental encryption methods: symmetric and asymmetric.
There are two types of encryption:
Symmetric Encryption -
Asymmetric encryption
Let’s understand one by one, first symmetric encryption:
Symmetric Encryption
It uses only a single key for both encryption and decryption. The key must be kept secret by both parties. That’s the only flaw of this type of encryption: If the key is lost or in the wrong hands, an intruder can easily access data.
How it works:
The sender encrypts the message using a secret key.
The receiver decrypts the message using that key only.
If a third party intercepts the communication and does not have the secret key, they can’t read messages.
It is speedy and efficient, which makes it ideal for encrypting large amounts of data.
However, as mentioned previously, storing and sharing that secret key securely is important.
You can try out this by using the Crypto library in JavaScript using the AES-256-CTR algorithm:
const crypto = require('crypto');
// Generate a random key for symmetric encryption
const secretKey = crypto.randomBytes(32); // 256-bit key
const algorithm = 'aes-256-ecb'; // Using ECB mode to avoid IV for simplicity
// Function to encrypt a message
function encrypt(text) {
const cipher = crypto.createCipher(algorithm, secretKey);
let encrypted = cipher.update(text, 'utf8', 'hex');
encrypted += cipher.final('hex');
return encrypted;
}
// Function to decrypt a message
function decrypt(encryptedText) {
const decipher = crypto.createDecipher(algorithm, secretKey);
let decrypted = decipher.update(encryptedText, 'hex', 'utf8');
decrypted += decipher.final('utf8');
return decrypted;
}
// Encrypt a message
const message = "This is a secret message";
const encryptedMessage = encrypt(message);
console.log("Encrypted Message:", encryptedMessage);
// Decrypt the message
const decryptedMessage = decrypt(encryptedMessage);
console.log("Decrypted Message:", decryptedMessage);
You will get this in the GitHub repo here.
Asymmetric Encryption
Unlike symmetric encryption, asymmetric encryption uses two keys: a public key for encryption and a private one for decryption. The public key can be shared openly, while the owner keeps the private key secret. This type of encryption is computationally more complex and slower than symmetric encryption, but it solves the key-sharing problem of symmetric encryption.
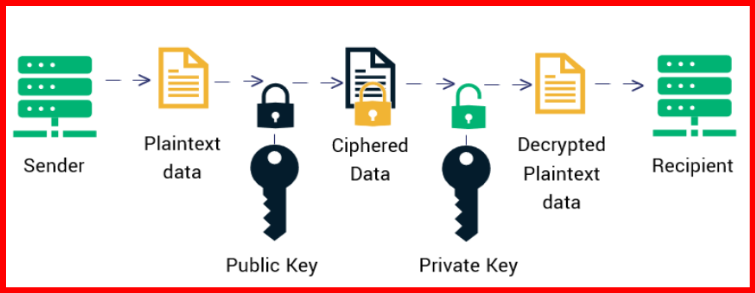
How Asymmetric Encryption Works:
The sender encrypts the message using the recipient’s public key.
The encrypted message is sent to the recipient.
The recipient decrypts the message using their private key.
Here is an example code you can try this out :
const crypto = require('crypto');
// Generate public and private keys
const { publicKey, privateKey } = crypto.generateKeyPairSync('rsa', { modulusLength: 2048, // RSA key length
});
// Function to encrypt a message using the public key
function encryptWithPublicKey(message) {
const encrypted = crypto.publicEncrypt(publicKey, Buffer.from(message));
return encrypted.toString('hex');
}
// Function to decrypt a message using the private key
function decryptWithPrivateKey(encryptedMessage) {
const decrypted = crypto.privateDecrypt(privateKey, Buffer.from(encryptedMessage, 'hex'));
return decrypted.toString();
}
// Encrypt a message
const secretMessage = "This is a top secret message";
const encryptedMessage = encryptWithPublicKey(secretMessage);
console.log("Encrypted Message:", encryptedMessage);
// Decrypt the message
const decryptedMessage = decryptWithPrivateKey(encryptedMessage);
console.log("Decrypted Message:", decryptedMessage);
How SSL Combines Symmetric and Asymmetric Encryption
Now we understand what are both doing, how things are working, and how these things are used in SSL certificates and use both of them -
SSL uses both symmetric and asymmetric encryption to provide secure communication to optimize performance. Here is how we are using these-
SSL Handshake using Asymmetric Encryption
When a browser connects to a web server, the SSL handshake begins. During the handshake, the web server shares its public key with the Browser, then the browser uses that public key to encrypt a symmetric key and send it back to the web server, and then the web server decrypts that using the private key. What’s the need for symmetric, then, if asymmetric works? As I said previously, symmetric is more accessible and efficient for large amounts of data. By using asymmetric at first, we share secret keys securely.
Data Transmission using Symmetric Encryption:
After the handshake, the client and server use the symmetric key to encrypt and decrypt data during the session. This approach is more efficient because symmetric encryption is faster and consumes fewer computational resources than asymmetric encryption.
Let’s break this whole process into steps-
To understand how SSL establishes a secure connection, let’s walk through the steps of the SSL handshake:
Browser Hello: The browser sends a "hello" message to the web server, including the SSL version and cryptographic settings it supports.
Web Server Hello: The web server responds with its "hello" message, choosing the best cryptographic settings from the client’s list. It also sends its SSL certificate, which contains its public key.
Certificate Verification: The browser verifies the web server’s certificate against trusted Certificate Authorities (CAs) to ensure the web server’s authenticity. (in the following points, we will understand what they are CAs)
.
Key Exchange: The browser generates a session key (symmetric key), encrypts it with the server’s public key, and sends it to the web server.
Session Key Decryption: The web server decrypts the session key using its private key. Now, both the browser and web server have the same session key.
Encrypted Communication: From this point onward, the session key is used to encrypt and decrypt all communication between the browser and web server.
SSL Certificates: Types and Levels of Trust
Now, Let’s Start with SSL certificates:
What are these? You are reading this on the DevRelSquad website. Let’s look practically.
Go To “view site information” -
Then click on the “connection is secure” button to view details.
Then, a goto certificate is a valid option.
Now, you will get an SSL certificate of the Devrel Squad website like this -
Types of Certificates
Domain Validated (DV) Certificates: These are the most basic certificates, providing validation only for the domain. They are typically used for personal websites and blogs.
Organization Validated (OV) Certificates: OV certificates provide higher trust by validating the organization behind the website. This type of certificate is ideal for business websites.
Extended Validation (EV) Certificates: EV certificates offer the highest level of security and trust. Websites with EV certificates display a green address bar in browsers, indicating a highly trusted connection.
Wildcard Certificates: Wildcard certificates secure a domain and all its subdomains (e.g., *.example.com). They are helpful for large organizations with multiple subdomains.
Multi-Domain (SAN) Certificates: These certificates can secure multiple domains under one certificate. Businesses with various websites often use them.
Different Types of Certificates used:
1. Root Certificate Authority certificate
2. Intermediate Certificate Authority Certificate
3. SSL certificate
Chain of Trust and How Certificates Work Together
The Root Certificate Authority (Root CA) is a top-level entity in the SSL ecosystem. It issues intermediate certificates, which are SSL certificates used by websites. The Root Certificate verifies the Intermediate Certificate by checking its digital signature, and then the intermediate certificate authority verifies the SSL certificate by validating its signature.
This whole process is known as the chain of trust.
If any certificate in this chain fails verification, the trust breaks and your browser will display a warning or prevent a secure connection. That’s why each link in the chain is essential for establishing a secure connection between your browser and the website.
What's Next?
In the next part of this series, we'll explore how to generate your own SSL certificate and test it on your local environment. This will include developing a self-signed certificate, understanding key pairs, and testing the SSL setup locally.
We'll also explain how different certificate levels work, including:
Self-Signed Certificates: Certificates signed by the entity (often used in development).
Domain-Validated (DV) Certificates: Used for basic website encryption.
Organization-Validated (OV) and Extended Validation (EV) Certificates: Offering a higher level of trust for more secure environments.
Stay tuned for Part 2, where you'll get hands-on with SSL certificate generation and local testing!
Output:
Source Code
You can find all the source code discussed in this article here on GitHub.
Great job breaking down SSL! This was super clear and helpful.
Really informative to read it sir!!
Great one I must say
Didn't know SSL certificates were this interesting, kudos brother👏
goooddd one sir thanks for the valueable infoo