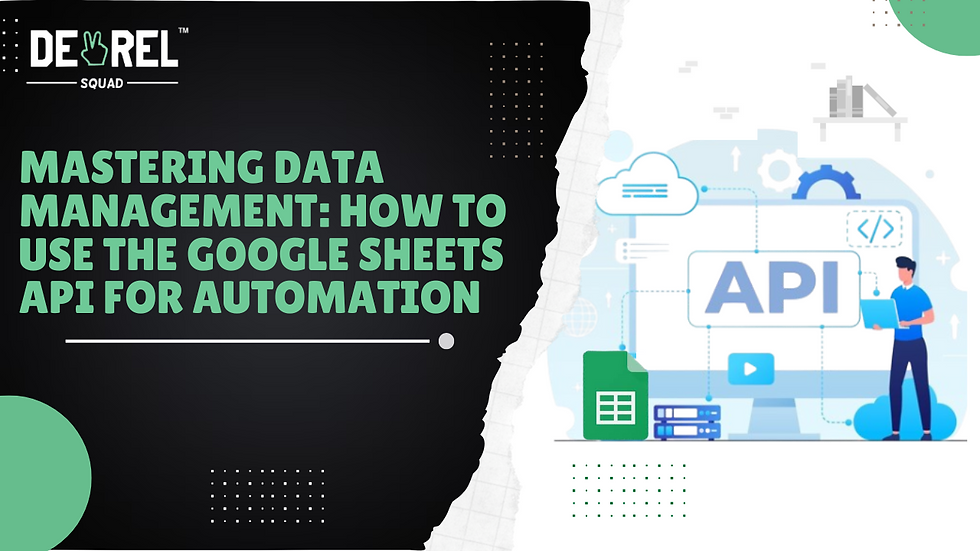
Utilize the Google Sheets API to maximize the functionality of Google Sheets. Learn how to automate importing, exporting, manipulating, and analyzing data. Discover practical uses, best practices, and advanced techniques to streamline operations and improve effectiveness.
Overview
Efficient data management is crucial for all types of businesses in today's data-driven environment. Google Sheets is widely used for managing data because of its user-friendly interface and efficient collaboration features. However, manually updating and managing large datasets can be time-consuming and prone to errors. The Google Sheets API offers a way to simplify different tasks performed in Google Sheets. This article will explore how to efficiently handle data using the Google Sheets API to automate processes on the Google Cloud Platform.
What is the Google Sheets API?
The Google Sheets API is a REST API that enables you to interact with your Google Sheets through code. It offers techniques for accessing, editing, and modifying data and for handling the layout and presentation of your documents. Utilizing this API allows for automating recurring tasks, connecting Google Sheets to different apps, and building interactive data workflows.
Why Use the Google Sheets API for Automation?
Enhancing productivity: Automating routine tasks helps save time and minimizes the chance of human mistakes.
Scalability: Handling and controlling vast amounts of data without being restricted by manual methods.
Integration: Incorporate Google Sheets with various tools and services to improve your data workflows seamlessly.
Real-time Updates: Receive automatic updates on your sheets with real-time information from different sources.
Getting Started with the Google Sheets API
Step 1: Set Up Your Google Cloud Project
The initial step in utilizing the API is setting up a Google Cloud project and activating the Google Sheets API.
Create a New Project: Head to the Google Cloud Console, initiate a new project and assign it a name.
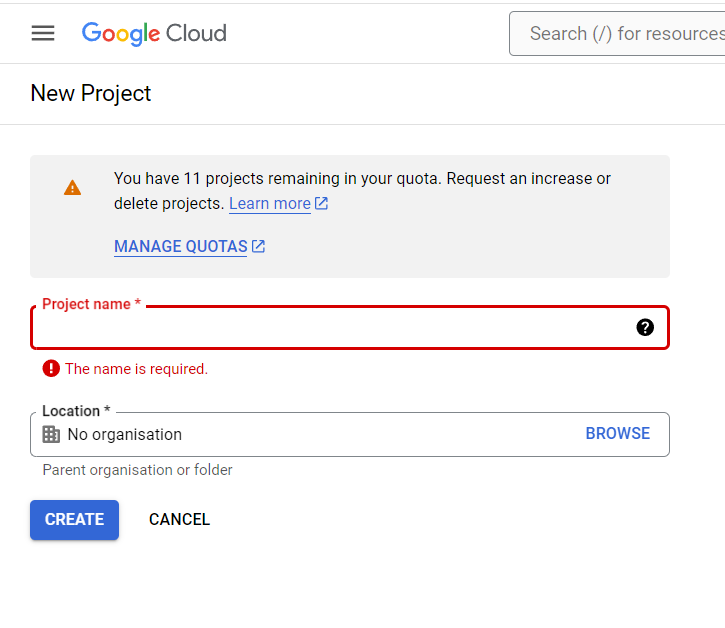
Enable the Google Sheets API: Activate the Google Sheets API by accessing the Cloud Console, going to "APIs & Services" > "Library", and looking up the "Google Sheets API". Select it and activate the API for your project.
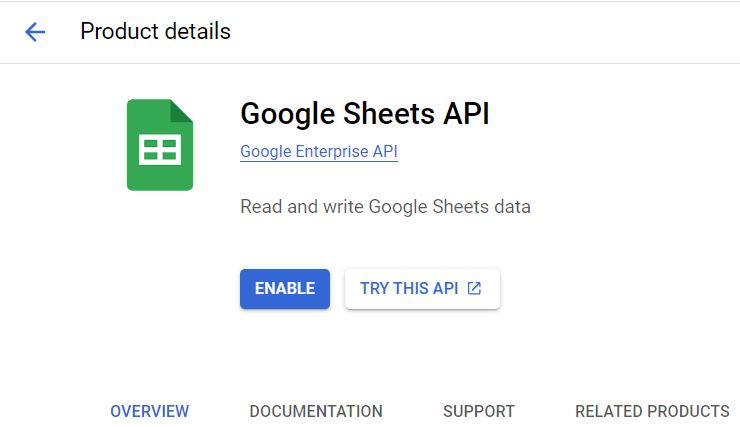
Create Credentials: To verify your requests, you must create credentials. Navigate to "APIs & Services" > "Credentials", press "Create Credentials", and choose "Service Account".
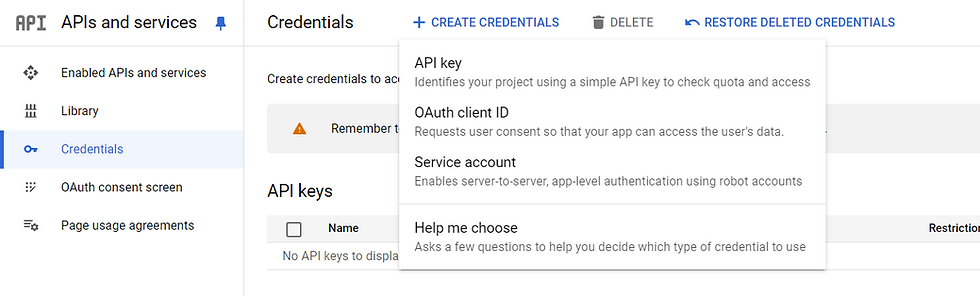
Step 2: Install the Google Client Library
You must install the Google client library based on your programming language. In this instance, Python will be utilized as a demonstration.
pip install --upgrade google-api-python-client google-auth-httplib2 google-auth-oauthlib
Step 3: Authenticate and Authorize
Using OAuth 2.0, you can authenticate and authorize your application to access the Google Sheets API.
from google.oauth2 import service_account
from googleapiclient.discovery import build
SCOPES = ['https://www.googleapis.com/auth/spreadsheets']
SERVICE_ACCOUNT_FILE = 'path/to/your/service-account-file.json'
credentials = service_account.Credentials.from_service_account_file(
SERVICE_ACCOUNT_FILE, scopes=SCOPES)
service = build('sheets', 'v4', credentials=credentials)
Step 4: Interacting with Google Sheets
You can now interact with your Google Sheets with the service object created.
Reading Data
You need the spreadsheet ID and the range of cells you want to read to read data from a Google Sheet.
SPREADSHEET_ID = 'your-spreadsheet-id'
RANGE_NAME = 'Sheet1!A1:D10'
result = service.spreadsheets().values().get(spreadsheetId=SPREADSHEET_ID, range=RANGE_NAME).execute()
values = result.get('values', [])
if not values:
print('No data found.')
else:
for row in values:
print(row)
Writing Data
To write data to a Google Sheet, specify the range and the values you want to insert.
values = [
["Item", "Cost", "Stock"],
["Apple", "1.00", "100"],
["Banana", "0.50", "150"],
]
body = {
'values': values
}
result = service.spreadsheets().values().update(
spreadsheetId=SPREADSHEET_ID, range=RANGE_NAME,
valueInputOption='RAW', body=body).execute()
print('{0} cells updated.'.format(result.get('updatedCells')))
Output:
Conclusion
Improving your productivity and organizing your workflows can significantly improve by mastering data management using the Google Sheets API. You can concentrate on more strategic aspects of your job by automating repeated tasks, connecting with other tools, and guaranteeing immediate data updates. Begin exploring the capabilities of the Google Sheets API now to maximize the efficiency of handling data on the Google Cloud Platform.
FAQs
1. What is the Google Sheets API?
The Google Sheets API is a RESTful tool that enables automated interaction with your Google Sheets. It offers techniques for accessing, modifying, and maintaining data, along with controlling the layout and appearance of your documents.
2. Why should I use the Google Sheets API for automation?
Employing the Google Sheets API for automation can result in time savings, error reduction, improved management of large datasets, and seamless integration with various tools and services.
3. How do I get started with the Google Sheets API?
Create a Google Cloud project, activate the Google Sheets API, and generate credentials. Next, download the Google client library and authorize your application to begin working with Google Sheets.
4. What are some advanced techniques for using the Google Sheets API?
Advanced techniques include updating data in batches, validating data, applying conditional formatting, and establishing triggers and webhooks for instant updates.
5. Can I integrate Google Sheets with other applications using the API?
Utilizing the Google Sheets API allows you to smoothly incorporate Google Sheets with other tools and services, thereby improving your data processes.
References:
Feel free to use the reference links for more detailed information and guides: Google Sheets API
Check out the GitHub repository for detailed source code and functional examples. Explore practical implementations to enhance your projects.
ความคิดเห็น