This guide will walk you through the steps to integrate Music into Our Application using Spotify Web API.
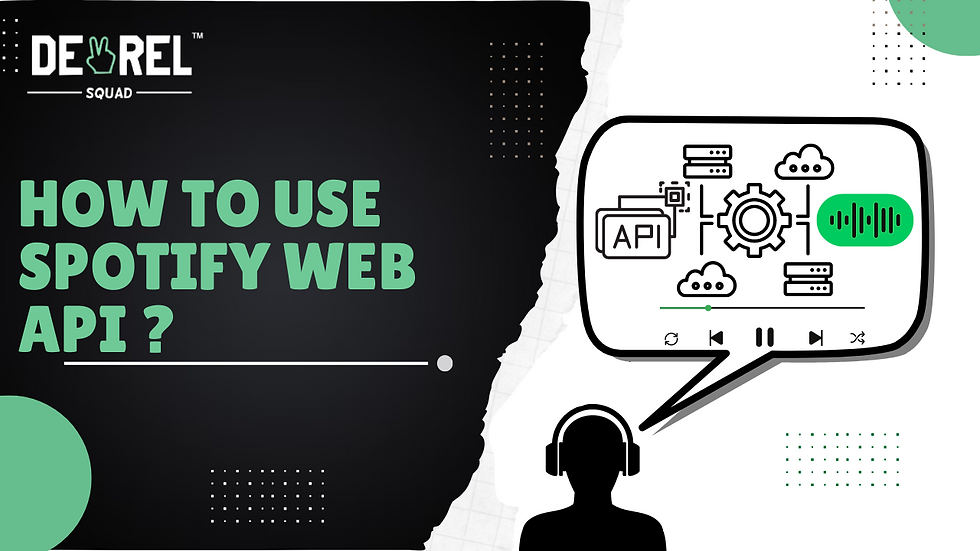
Overview
Using some of Spotify's features can improve your application's user experience. The robust Spotify Web API allows developers to access and modify user playlists, search songs and albums, and perform many other operations by interacting with Spotify's database.
In this article, we will learn how to access the Spotify Web API, authenticate requests, and handle music data in our application.
What is Spotify Web API?
The RESTful Spotify Web API provides access to Spotify's music catalogue and user data. It lets developers obtain complete data about songs, albums, artists, playlists, and other Spotify properties.
Setting Up Spotify Web API
Step 1: Create a Spotify Developer Account
To use the Spotify Web API, you first need to create a Spotify Developer Account:
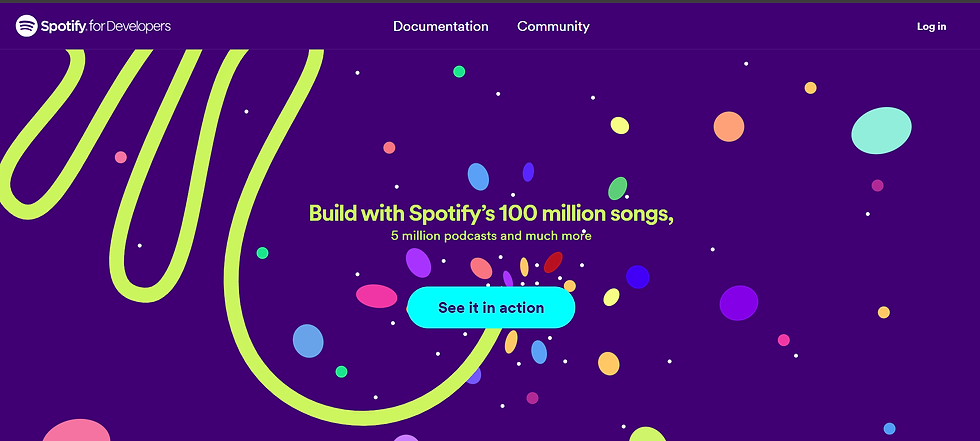
2. Login with your Spotify account or create a new one.
3. Click on Create an App.
4. Fill in the required details and click Create.
Step 2: Get Your Client ID and Client Secret
After generating your app, you will receive a Client ID and Client Secret. These are necessary for sending authorized requests to the Spotify Web API.
Step 3: Set Up Redirect URI
Let's set up a Redirect URI for OAuth authentication:
Click on Edit Settings in your app dashboard.
Add your redirect URI under Redirect URIs.
Click Save.
Step 4: Authentication
Spotify uses OAuth for authentication. To access Spotify's data, your application must be authenticated.
Python
import requests
import base64
def get_token(client_id, client_secret):
auth_str = f"{client_id}:{client_secret}"
b64_auth_str = base64.b64encode(auth_str.encode()).decode()
headers = {
"Authorization": f"Basic {b64_auth_str}"
}
data = {
"grant_type": "client_credentials"
}
response = requests.post("https://accounts.spotify.com/api/token", headers=headers, data=data)
response_data = response.json()
return response_data["access_token"]
client_id = "your_client_id"
client_secret = "your_client_secret"
access_token = get_token(client_id, client_secret)
print(f"Access Token: {access_token}")
This script requests a POST to Spotify's Accounts Service to obtain an OAuth token using your client credentials.
The returned token is essential for authenticating subsequent API requests.
Using Spotify Web API
Fetching Data Example in Python
Here is an example in Python that shows you how to use the Spotify Web API to retrieve track data:
import requests
def get_track(track_id, access_token):
headers = {
"Authorization": f"Bearer {access_token}"
}
response = requests.get(f"https://api.spotify.com/v1/tracks/{track_id}", headers=headers)
return response.json()
# Example
track_id = "3n3Ppam7vgaVa1iaRUc9Lp"
track_data = get_track(track_id, access_token)
print(track_data)
Using the supplied track ID, this script makes a GET request to the Spotify Web API to retrieve information about a particular music.
The function authenticates the request by using the OAuth token that was previously acquired.
Fetching Data Example in JavaScript
You can utilize the Spotify Web API in JavaScript for web apps. Let's observe this simple example:
The Spotify Web API client library should first be included in your HTML:
<script src="https://apis.google.com/js/api.js"></script>
Next, add the following JavaScriptcode to fetch data:
async function getToken() {
const client_id = 'your_client_id';
const client_secret = 'your_client_secret';
const url = 'https://accounts.spotify.com/api/token';
const headers = new Headers();
headers.append('Content-Type', 'application/x-www-form-urlencoded');
headers.append('Authorization', 'Basic ' + btoa(client_id + ':' + client_secret));
const body = new URLSearchParams();
body.append('grant_type', 'client_credentials');
const response = await fetch(url, {
method: 'POST',
headers: headers,
body: body
});
const data = await response.json();
return data.access_token;
}
async function getTrack(track_id) {
const token = await getToken();
const url = `https://api.spotify.com/v1/tracks/${track_id}`;
const response = await fetch(url, {
headers: {
'Authorization': 'Bearer ' + token
}
});
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
const data = await response.json();
return data;
}
// Example
getTrack('3n3Ppam7vgaVa1iaRUc9Lp')
.then(track => {
console.log(track);
})
.catch(error => {
console.error('Error fetching track:', error);
});
This JavaScript code authenticates the application and fetches track data by sending a GET request to the Spotify Web API.
The OAuth token is obtained in the getToken function and is used to authenticate the request in the getTrack function.
Handling Errors in JavaScript
In Our program, handling mistakes is essential. An improved JavaScript method with fundamental error handling is shown below:
async function getToken() {
// Token generation code
// ...
}
async function getTrack(track_id) {
try {
const token = await getToken();
const url = `https://api.spotify.com/v1/tracks/${track_id}`; const response = await fetch(url, {
headers: {
'Authorization': 'Bearer ' + token
}
});
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`); }
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error occurred:', error);
}
}
getTrack('3n3Ppam7vgaVa1iaRUc9Lp');
The getTrack function now includes a try-catch block to handle errors during the fetch request.
If an error occurs, it is logged to the console with a descriptive message.
Output
Why Use Spotify Web API?
The following are solid reasons for utilizing the Spotify Web API:
Access Rich Music Data: Quickly browse an extensive database of songs, playlists, albums, and artists.
Customize User Experience: Personalize the experience based on the user's listening preferences and history.
Simple Integration: The RESTful API architecture facilitates integration.
Scalability: The API is suitable for projects of any size and can handle a high rate of inquiries.
Innovation: Use powerful music features to enrich your app.
Conclusion
Simplify the process of incorporating Spotify's music catalogue into our applications.
It is simple to set up, Manage playlists, and obtain comprehensive music information in simple steps.
FAQs
1. What are the costs associated with Spotify Web API?
The Spotify Web API is free to use. However, it comes with rate limits, which can be found on the Spotify Developer website.
2. What data can I access using the Spotify Web API?
The Spotify Web API allows us to access a wide range of data, including track information, playlists, albums, artists, and user data (with appropriate permissions).
3. How does Spotify guarantee that the information is accurate?
Spotify ensures that developers can access accurate and current data by regularly updating its database with the most up-to-date song information.
References
Feel free to use the reference links for more detailed information and guides:
Explore this GitHub repository for source code and examples to enrich your projects with Spotify Web API integration.
Happy coding!✨🚀
Great blog! The step-by-step guide on integrating the Spotify Web API was really easy to follow. Thankyou for this helpful post 🙌🏻
Very detailed explanations, well done
Well explained 🙌✨