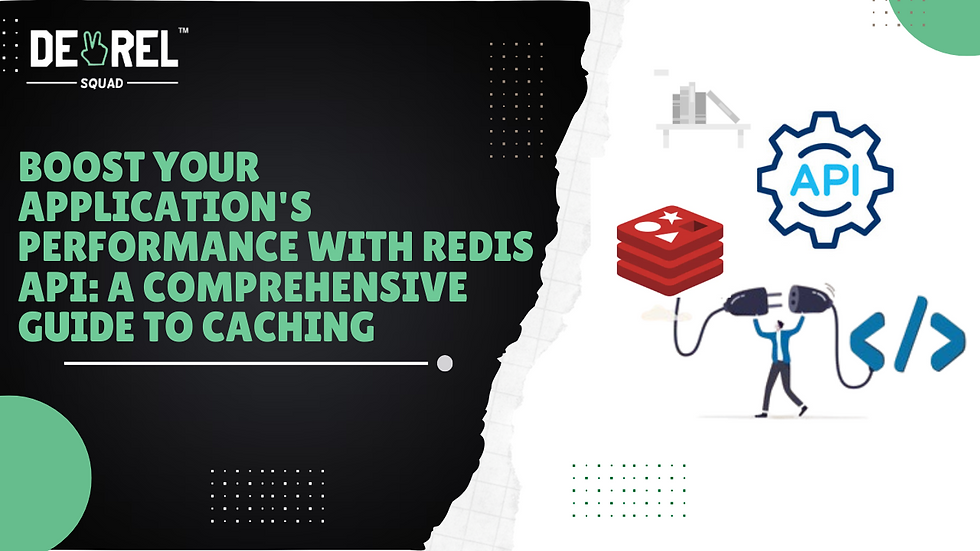
Overview
In today's fast-paced digital world, application performance is crucial. Caching is an essential optimization technique that stores frequently accessed data in memory, reducing latency and server load by avoiding repeated retrieval from slower sources like databases or APIs. This significantly improves response times and overall application speed.
This blog will guide you through integrating Redis into your application using the Redis API, specifically focusing on a Golang implementation.
What is Redis API?
Redis is an open-source, in-memory data structure store that excels as a caching layer. There are various reasons to use Redis for caching your data:
High-Performance Speed: Redis stores data using in-memory operations, making it as fast as lightning McQueen for read-write operations, even in traditional databases.
Scalability: Redis can be scaled horizontally by adding more nodes to the cluster.
Rich Data Structures: Redis offers various data structures like Hashes, Lists, Sets, and Sorted Sets. This allows you to store and retrieve data efficiently based on the use case.
Persistence: While primarily in-memory, Redis can persist data to disk for data recovery in case of restarts.
How to Implement the Redis in Your Go Application
In this example, we will create a simple Go Web Server, connect it to our Redis instance, and implement the caching for a simple HTTP GET Request.
Download or Clone the code sample for the Web Server program in Go that uses Redis to implement the Caching.
Prerequisite
The latest version of Go is installed on your machine.
Step 1: Initialize a Go Application
Create a folder and initialize your Go application by running the following command.
go mod init myWebServer
This will create a "go.mod" file in your code folder.
Now, create a file named "main.go" in your root folder.
Step 2: Setup the Go Server
In the "main.go" file, create a simple web server that will serve the HTTP GET request. To achieve this, we will import "net/http" package from the standard library of Go.
Create a function, say "getData()" (we will use this function to write the code to fetch the data.) A sample structure of our web server
In this web server, we have defined the API endpoint "/api/devrelData" and the port is 8080.
package main
import (
"fmt"
"net/http"
)
func main() {
fmt.Println("A Sample Web Server with Redis Caching")
http.HandleFunc("/api/devrelData", getData)
http.ListenAndServe(":8080", nil)
}
func getData(w http.ResponseWriter, r *http.Request) {
// we will fetch our data here
fmt.Fprintf(w, "Hello fellow DevRel's")
}
Step 3: Connecting to Redis Instance
Now, you will connect our web server to the Redis instance in this step. To achieve this, you need to install Redis Client on your local machine using the "go-redis" package.
go get github.com/redis/go-redis/v9

Now import this package and establish a connection to your Redis instance
We will use "redisClient" variable to interact with Redis by defining the host address (Addr), your password (Password) and the index of the database to be selected after connecting to the server (DB)
import (
"context"
"fmt"
"net/http"
"github.com/redis/go-redis/v9"
)
var ctxBg = context.Background()
var redisClient *redis.Client;
func main() {
fmt.Println("A Sample Web Server with Redis Caching")
// initialize the redis client
redisClient = redis.NewClient(&redis.Options{
Addr: "localhost:<PORT_NUMBER>",
Password: "",
DB: 0,
})
http.HandleFunc("/api/devrelData", getData) //defining the api endpoint
http.ListenAndServe(":8080", nil) //defining the port
}
Step 4: Implementing Caching with Redis
We will now implement caching for the "/api/devrelData" HTTP GET request. The data will be saved with a cache key and an expiration time. We'll first see if the cache key exists in Redis when the same request comes in. If it does, we'll return the cached data; otherwise, we'll get it, store it in Redis, and send it back to the client.
We will implement this cache logic in our "getData()" function that we created in the Step 2
package main
import (
"context"
"fmt"
"net/http"
"time"
"github.com/redis/go-redis/v9"
)
//...
func getData(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello fellow DevRel's")
// code to fetch the data
cacheKey := "api-data-cache"
// check if cache key exists in Redis instance
cachedData, err := redisClient.Get(ctxBg, cacheKey).Result()
// error handling
if err == redis.Nil{
// if cache key not found, fetch and store the data in Redis
data := "Hello Aman"
cacheExpiration := 10*time.Minute
err := redisClient.Set(ctxBg, cacheKey, data, cacheExpiration).Err()
if err!=nil {
panic(err)
}
fmt.Fprintf(w, data)
}else if err!=nil {
panic(err)
}else {
// cache key found, hence return this data
fmt.Fprintf(w, cachedData)
}
}
Step 5: Testing the Application
Now, send a GET request to "http://localhost:8080/api/devrelData". You can use Postman or the cURL command for this.
The first request will fetch the data and store it in Redis. Subsequent requests within the cache expiration time will return the cached data. You can also monitor the Redis instance using the "redis-cli monitor" command to see the cache-related operations in real-time.
Conclusion
Implementing Redis caching in your Go applications can significantly boost performance, reduce the load on your primary database, and improve response times. This simple example demonstrates the basics, but Redis offers much more. As you build more complex applications, explore Redis's advanced features like pipelining, transactions, and pub/sub messaging to optimize your caching strategy further.
Remember, while caching is powerful, it also introduces complexity. Consider the trade-offs and ensure your caching logic maintains data consistency and handles edge cases appropriately.
FAQs
Q. Do I need to know Go programming to understand this guide?
A. The concepts can be applied to other programming languages, though the guide implements Go. But you'll find it easier to follow the code examples if you are familiar with Go.
Q: How can I test if my caching is working?
A: You can test by sending repeated requests to your application and observing if subsequent requests are faster due to cached data.
Q: Can Redis persist data, or is it only in memory?
A: While Redis is primarily an in-memory store, it can also persist data in a disk for recovery in case of restarts.
Q: How do I handle cache expiration in Redis?
A: Redis allows you to set an expiration time when storing data. In the guide's example, this is done using the cacheExpiration variable when setting data in Redis
Comments